Alright guyssss … welcome back to our channel… hahah seperti biasa kita lanjutkan lagi sedikit materi Svelte kita …
Clock.svelte
<script>
import { onMount } from 'svelte';
let time = new Date();
// these automatically update when `time`
// changes, because of the `$:` prefix
$: hours = time.getHours();
$: minutes = time.getMinutes();
$: seconds = time.getSeconds();
onMount(() => {
const interval = setInterval(() => {
time = new Date();
}, 1000);
return () => {
clearInterval(interval);
};
});
</script>
<svg viewBox='-50 -50 100 100'>
<circle class='clock-face' r='48'/>
<!-- markers -->
{#each [0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55] as minute}
<line
class='major'
y1='35'
y2='45'
transform='rotate({30 * minute})'
/>
{#each [1, 2, 3, 4] as offset}
<line
class='minor'
y1='42'
y2='45'
transform='rotate({6 * (minute + offset)})'
/>
{/each}
{/each}
<!-- hour hand -->
<line
class='hour'
y1='2'
y2='-20'
transform='rotate({30 * hours + minutes / 2})'
/>
<!-- minute hand -->
<line
class='minute'
y1='4'
y2='-30'
transform='rotate({6 * minutes + seconds / 10})'
/>
<!-- second hand -->
<g transform='rotate({6 * seconds})'>
<line class='second' y1='10' y2='-38'/>
<line class='second-counterweight' y1='10' y2='2'/>
</g>
</svg>
<style>
svg {
width: 100%;
height: 100%;
}
.clock-face {
stroke: #333;
fill: white;
}
.minor {
stroke: #999;
stroke-width: 0.5;
}
.major {
stroke: #333;
stroke-width: 1;
}
.hour {
stroke: #333;
}
.minute {
stroke: #666;
}
.second, .second-counterweight {
stroke: rgb(180,0,0);
}
.second-counterweight {
stroke-width: 3;
}
</style>
dan import ke App.svelte
<main>
<h1>Hello {name}!</h1>
<p>Visit the <a href="https://svelte.dev/tutorial">Svelte tutorial</a> to learn how to build Svelte apps.</p>
<Counter />
<Toggle />
<Img />
<String />
<Statement />
<Condition />
<Nested answer={42}/>
<Nested/>
<Info {...pkg}/>
<IfLogin />
<Comparison />
<EachYoutube />
<KeyEachThing />
<GenerateNumber />
<MouseMove />
<MouseMoveInlineHandler />
<EventModifier />
<AlertCompEvent />
<AlertEventForwarding />
<CustomButtonComp />
<TextInput />
<NumericInput />
<CheckboxInputs />
<GroupInputs />
<TextareaInputs />
<FileInput />
<SelectBindings />
<SelectMultiple />
<EachBlockBindings />
<MediaElements />
<Dimensions />
<BindThisCanvas />
<ComponentBindings />
<OnMount />
<OnDestroyIntervals />
<BeforeAndAfterUpdate />
<Tick />
<h1>The count is {countValue}</h1>
<Incrementer/>
<Decrementer/>
<Resetter/>
<h1>The count is {$countAutoSubscriptions}</h1>
<IncrementerAutoSubscriptions/>
<DecrementerAutoSubscriptions/>
<ResetterAutoSubscriptions/>
<h1>The time is {formatter.format($time)}</h1>
<h1>The time is {formatter.format($time)}</h1>
<p>
This page has been open for
{$elapsed} {$elapsed === 1 ? 'second' : 'seconds'}
</p>
<h1>The count is {$countCustom}</h1>
<button on:click={countCustom.increment}>+</button>
<button on:click={countCustom.decrement}>-</button>
<button on:click={countCustom.reset}>reset</button>
<Tweened />
<Spring />
<TransitionsDirective />
<AddingParameters />
<InOut />
<CustomCss />
<CustomJs />
<TransitionEvents />
<Deffered />
<AnimateDirective />
<Visualiser />
<Clock />
</main>
hasilnya…
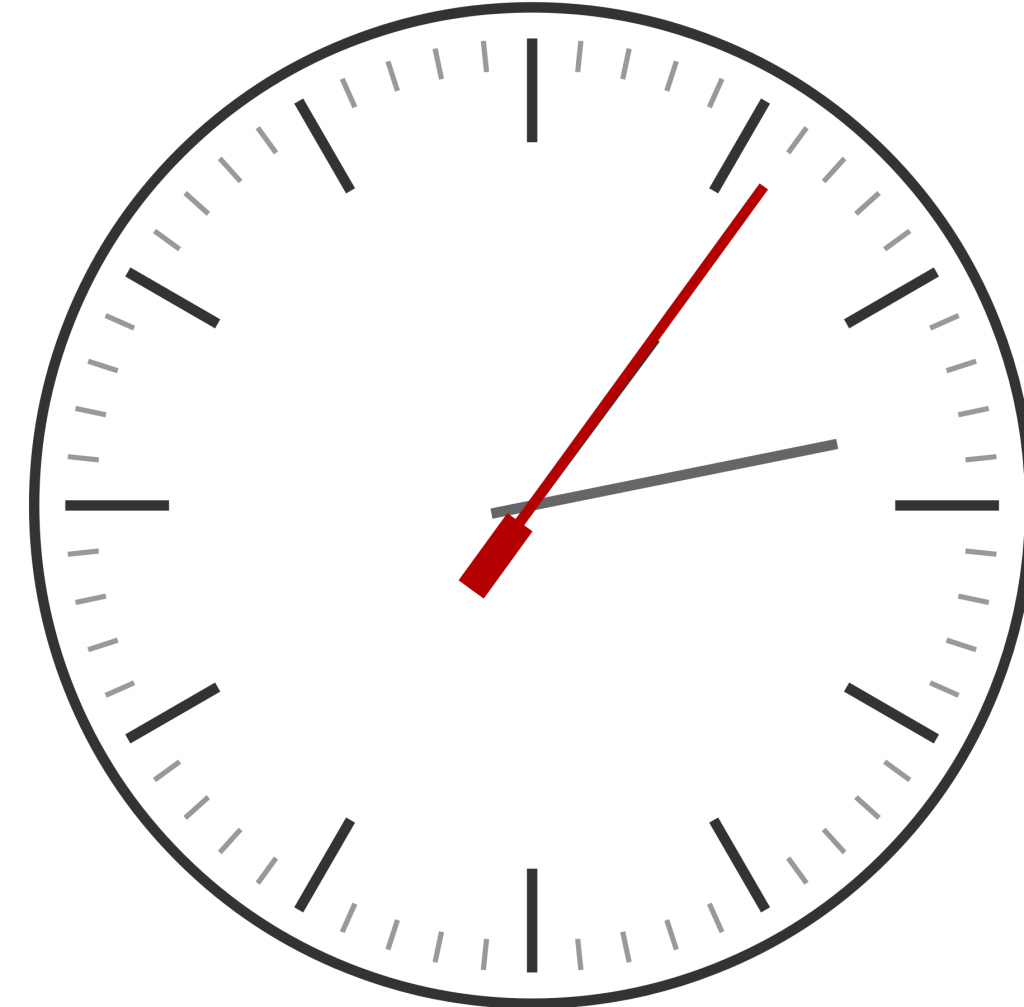
Okay berhasil yagesya…. link github ada disini dan sampai jumpa di post selanjutnya,… cyaaa….