ModalComp.svelte
<script>
import { createEventDispatcher, onDestroy } from 'svelte';
const dispatch = createEventDispatcher();
const close = () => dispatch('close');
let modal;
const handle_keydown = e => {
if (e.key === 'Escape') {
close();
return;
}
if (e.key === 'Tab') {
// trap focus
const nodes = modal.querySelectorAll('*');
const tabbable = Array.from(nodes).filter(n => n.tabIndex >= 0);
let index = tabbable.indexOf(document.activeElement);
if (index === -1 && e.shiftKey) index = 0;
index += tabbable.length + (e.shiftKey ? -1 : 1);
index %= tabbable.length;
tabbable[index].focus();
e.preventDefault();
}
};
const previously_focused = typeof document !== 'undefined' && document.activeElement;
if (previously_focused) {
onDestroy(() => {
previously_focused.focus();
});
}
</script>
<svelte:window on:keydown={handle_keydown}/>
<div class="modal-background" on:click={close}></div>
<div class="modal" role="dialog" aria-modal="true" bind:this={modal}>
<slot name="header"></slot>
<hr>
<slot></slot>
<hr>
<!-- svelte-ignore a11y-autofocus -->
<button autofocus on:click={close}>close modal</button>
</div>
<style>
.modal-background {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0,0,0,0.3);
}
.modal {
position: absolute;
left: 50%;
top: 50%;
width: calc(100vw - 4em);
max-width: 32em;
max-height: calc(100vh - 4em);
overflow: auto;
transform: translate(-50%,-50%);
padding: 1em;
border-radius: 0.2em;
background: white;
}
button {
display: block;
}
</style>
Modal.svelte
<script>
import ModalComp from './components/ModalComp.svelte';
let showModal = false;
</script>
<button on:click="{() => showModal = true}">
show modal
</button>
{#if showModal}
<ModalComp on:close="{() => showModal = false}">
<h2 slot="header">
modal
<small><em>adjective</em> mod·al \ˈmō-dəl\</small>
</h2>
<ol class="definition-list">
<li>of or relating to modality in logic</li>
<li>containing provisions as to the mode of procedure or the manner of taking effect —used of a contract or legacy</li>
<li>of or relating to a musical mode</li>
<li>of or relating to structure as opposed to substance</li>
<li>of, relating to, or constituting a grammatical form or category characteristically indicating predication</li>
<li>of or relating to a statistical mode</li>
</ol>
<a href="https://www.merriam-webster.com/dictionary/modal">merriam-webster.com</a>
</ModalComp>
{/if}
dan import ke App.svelte
</ContactCard>
<Hoverable />
<Profile />
<Modal />
dan hasilnya akan seperti ini …
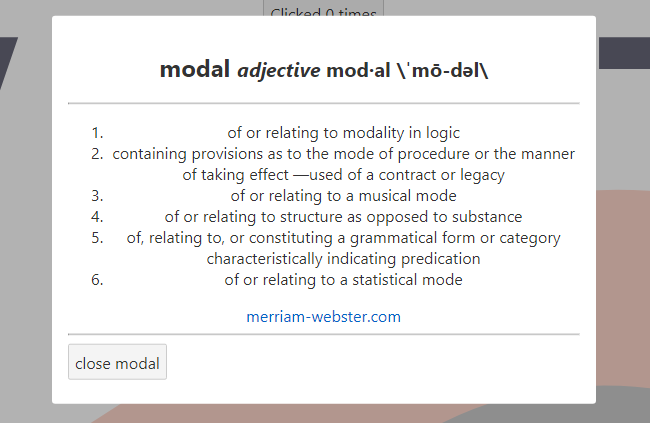
Allright berhasil ya… source code github ada disini… cyaaa